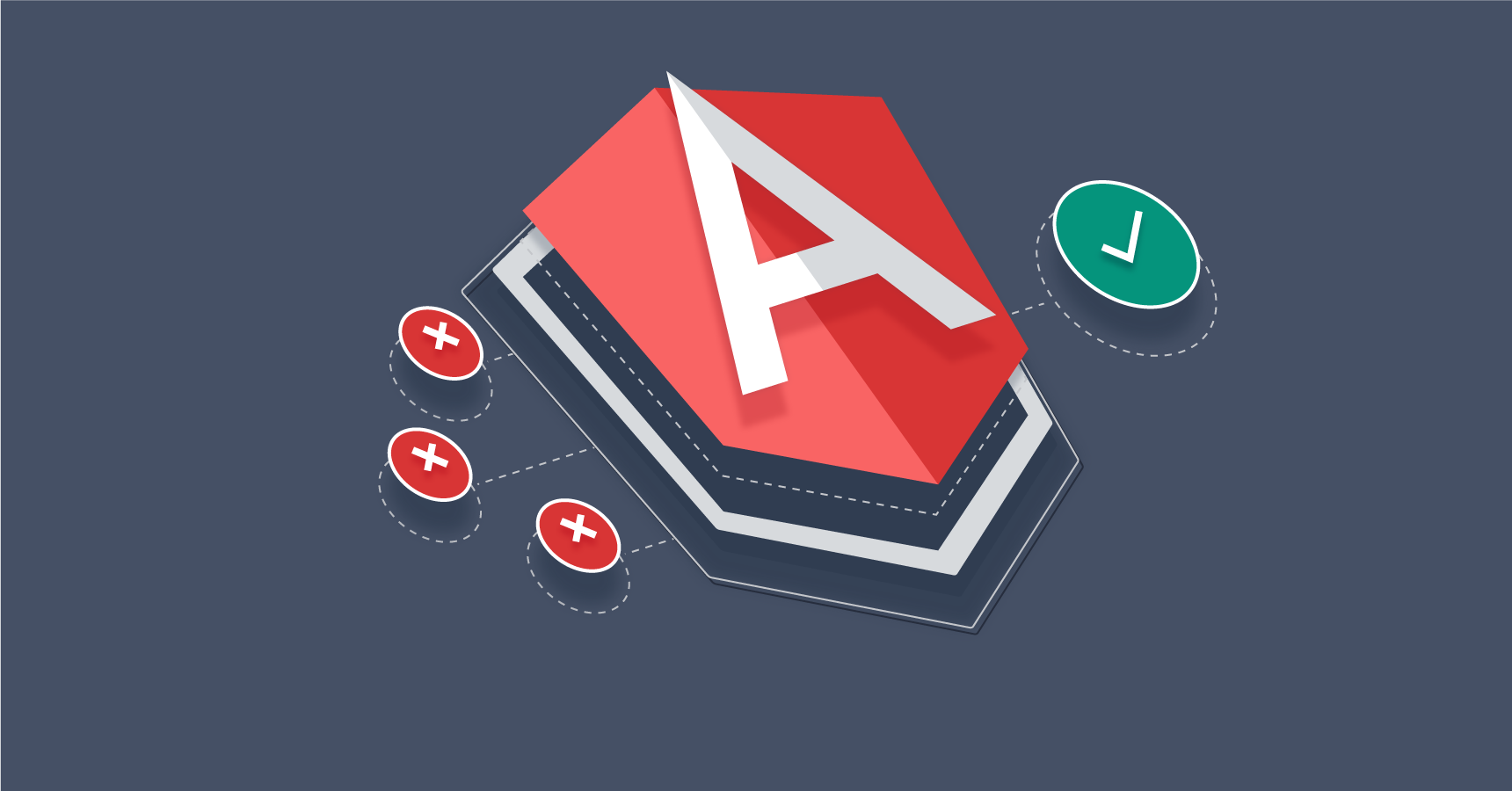
- Duration
5 Months
- Language
Hindi/English
- Certificate
Yes
- Expert Trainers
Yes
- Flexible Timing
Yes
- Fee Installements
Yes
- Communication Classes
Free
Description
A frontend angular developer implements web design to create an optimal interface through programming languages like HTML, CSS, and Javascript. Used for developing web, mobile, and desktop applications, the goal of this open-source framework is to bring simplification and structure to the JavaScript language. Angular is a JavaScript-based open-source front-end web framework for developing single-page applications. It was mainly maintained by Google and a community of individuals and corporations. Its aim was to simplify both the development and testing of such applications by providing a framework for client-side model–view–controller (MVC) architecture, along with components commonly used in web applications and progressive web applications. Additionally, OddIQ IT Institute specializes in providing exceptional software training and placement opportunities in Angular development.
Course Content Overview:
Introduction to Angular
• What is Angular?
• Angular Versions: AngularJS (vs) Angular
• Setup for local development environment
o Installing NodeJS, NPM
o Angular CLI
• Develop First Angular program using Angular CLI and Visual Studio Code.
Angular Architecture
• Introduction
• Basic Building Blocks of Angular Applications
• Angular Modules and @NgModule decorator
• Angular Libraries
• Component, Templates and Metadata
• Data Binding
• Directives
• Services and Dependency Injection
Displaying Dynamic Data
• Types of Directives
• Template Expressions
• String Interpolation
• Built-In Directives
o ngIf
o ngSwitch
o ngFor
• * vs <template>
• When to use <ng-container>
Angular Components Deep Dive
• What are Components?
• Components Life Cycle Hooks.
• Dynamic Components.
• Working with Model Class.
• Nested Components.
• Working with Arrays / Collections.
Data Binding
• Binding properties and Interpolation
• One-way Binding / Property Binding
• Event Binding
• Two-way Binding
• Two-way binding with NgModel
• Attribute Binding
• Style and Class Binding
Styles Binding In Components
• Style and Class Bindings
• Built-In Directives – NgStyle & NgClass
• Using Component Styles
• Special selectors
• Loading Styles into Components
• View Encapsulation
• ViewChild & ViewChildren
• ContentChild & ContentChildren
Template Driven Forms
• Introduction
• Create the component that controls the form
• Create a template with the initial form layout
• Bind data properties to each form input control with the ngModel two-way data binding syntax
• Add the name attribute to each form input control
• Add custom CSS to provide visual feedback
• Show and hide validation error messages
• Handle form submission with ngSubmit
• Disable the form’s submit button until the form is valid
• Resetting the form.
Reactive Forms
• Reactive Forms Introduction
• More Form Controls
• Form Control Properties
• setValue and patchValue
• Validating Form Elements
• Submitting and Resetting forms
• Observing and Reacting to Form Changes
Deccansoft Software Services Angular 7 Syllabus
• Using FormBuilder
Working with Pipes
• Built-in Pipes
• Using parameters and chaining Pipes
• Custom Pipes
• Parameterized Custom Pipe
• Pipes and Change Detection
• Pure and Impure pipes
• Changes in Pipes Syntax from 4 to 5
Custom Directives
• Custom Attribute Directive
• Using HostListener
• Using HostBinding
• Custom Validator Directive
Dependency Injection
• Understanding Dependency Injection
• Understanding DI in Angular Framework
• ReflectiveInjector
• Exploring Provider
• Types of Tokens
• Types of Dependencies
• Configuring DI using Providers
• Implementing DI in Angular
• Optional Dependencies
Services in Angular
• Building and Injecting Custom Services
• Service using another Service
Reactive Extension for JavaScript
• Introduction
• Observable and Observer
• Reactive Operators
Http Client Service
• HttpClientModule and HttpClient Classes
• Writing Service with Get / Add / Edit / Delete
• Using Service in Component
Angular Routing
• Introduction
• Configuring and Navigating
• Parameterized routes
• Nested (or) Child Routes
• Router Guards & Routing Strategies
Angular Modules
• AppModule as Root Module
• Feature modules
• Lazy Loading a Module
• Shared Module